How Developers Can Make improvements to Their Debugging Techniques By Gustavo Woltmann
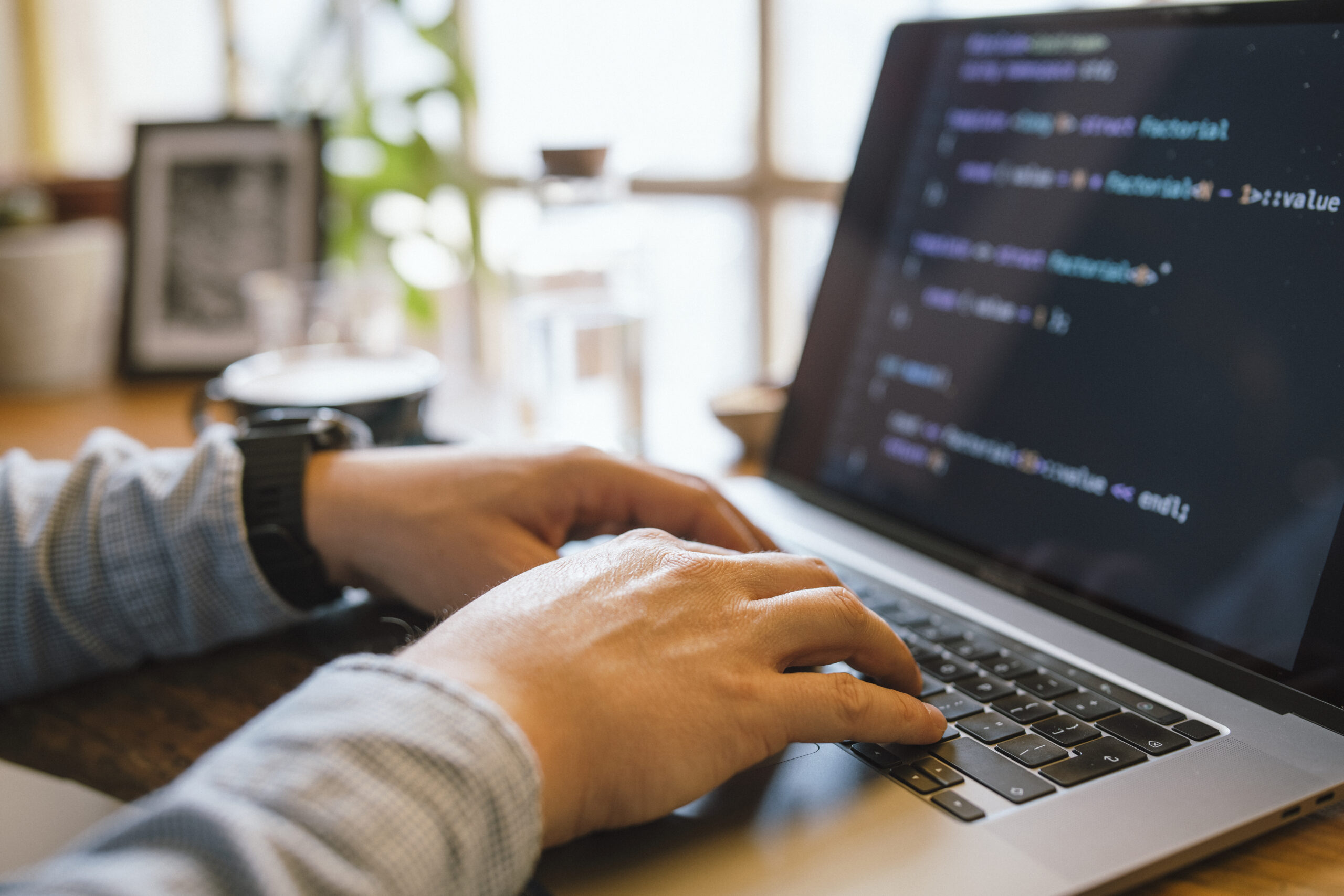
Debugging is Just about the most crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to Feel methodically to resolve troubles proficiently. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and significantly enhance your productivity. Listed below are numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Learn Your Applications
Among the list of fastest techniques developers can elevate their debugging skills is by mastering the resources they use every day. Though crafting code is a person Section of advancement, figuring out the way to interact with it correctly through execution is equally important. Fashionable development environments appear equipped with powerful debugging abilities — but numerous builders only scratch the area of what these equipment can perform.
Just take, as an example, an Built-in Advancement Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These equipment let you established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and also modify code on the fly. When utilised properly, they Enable you to observe particularly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They assist you to inspect the DOM, check community requests, see true-time performance metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can turn aggravating UI challenges into manageable duties.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating procedures and memory management. Understanding these instruments can have a steeper Studying curve but pays off when debugging functionality problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be aware of code background, locate the precise moment bugs had been launched, and isolate problematic variations.
In the long run, mastering your applications indicates going over and above default options and shortcuts — it’s about producing an personal understanding of your advancement natural environment to make sure that when issues arise, you’re not dropped at the hours of darkness. The higher you are aware of your applications, the greater time you could expend resolving the particular trouble rather then fumbling through the procedure.
Reproduce the condition
One of the most significant — and often ignored — actions in efficient debugging is reproducing the problem. Before leaping in the code or earning guesses, builders need to have to make a regular natural environment or circumstance exactly where the bug reliably appears. With out reproducibility, correcting a bug will become a match of likelihood, often bringing about squandered time and fragile code alterations.
Step one in reproducing a problem is accumulating as much context as possible. Check with issues like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise situations under which the bug happens.
After you’ve gathered adequate information, try and recreate the issue in your neighborhood environment. This may suggest inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem appears intermittently, look at writing automated assessments that replicate the edge circumstances or point out transitions involved. These checks not just support expose the problem but in addition reduce regressions in the future.
Often, the issue could possibly be ecosystem-particular — it would transpire only on certain working units, browsers, or under distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It needs persistence, observation, and also a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You need to use your debugging instruments additional correctly, exam opportunity fixes properly, and connect extra Obviously using your crew or end users. It turns an abstract complaint into a concrete challenge — Which’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Error messages are frequently the most worthy clues a developer has when a thing goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, in which it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information very carefully and in full. Lots of developers, especially when underneath time strain, look at the 1st line and promptly commence making assumptions. But further inside the mistake stack or logs could lie the true root bring about. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Is it a syntax error, a runtime exception, or perhaps a logic mistake? Does it position to a selected file and line variety? What module or function induced it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also beneficial to understand the terminology in the programming language or framework you’re applying. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some problems are imprecise or generic, As well as in These situations, it’s very important to examine the context through which the mistake happened. Check out related log entries, input values, and recent improvements during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s happening beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log get more info and at what level. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic data in the course of progress, Details for standard activities (like effective start-ups), Alert for probable troubles that don’t break the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital gatherings, state alterations, enter/output values, and critical conclusion factors in your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t probable.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-imagined-out logging solution, you may reduce the time it requires to identify challenges, obtain further visibility into your purposes, and improve the All round maintainability and dependability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To efficiently establish and resolve bugs, builders will have to approach the method just like a detective fixing a secret. This mindset assists break down elaborate problems into manageable elements and comply with clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the indicators of the situation: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much relevant info as it is possible to devoid of leaping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear picture of what’s happening.
Next, kind hypotheses. Request oneself: What could possibly be creating this behavior? Have any changes recently been made into the codebase? Has this challenge occurred before under identical situation? The purpose is always to narrow down possibilities and detect probable culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a controlled natural environment. In case you suspect a particular functionality or part, isolate it and verify if The difficulty persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest information. Bugs frequently disguise inside the the very least anticipated places—just like a missing semicolon, an off-by-one particular error, or maybe a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue without the need of completely being familiar with it. Short term fixes may conceal the actual issue, just for it to resurface afterwards.
And finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for future concerns and assist Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in advanced systems.
Compose Assessments
Crafting tests is one of the most effective strategies to help your debugging skills and General development efficiency. Exams not merely support capture bugs early but will also function a security Web that offers you confidence when creating variations to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically the place and when a difficulty happens.
Begin with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can promptly expose no matter if a certain piece of logic is Functioning as predicted. Each time a examination fails, you right away know in which to appear, considerably reducing some time used debugging. Device exams are Particularly handy for catching regression bugs—difficulties that reappear following previously remaining preset.
Following, integrate integration exams and end-to-end assessments into your workflow. These support make certain that many portions of your software function together efficiently. They’re specifically helpful for catching bugs that occur in advanced techniques with numerous factors or services interacting. If a thing breaks, your exams can tell you which Component of the pipeline failed and below what disorders.
Composing tests also forces you to definitely Believe critically regarding your code. To test a function adequately, you will need to know its inputs, predicted outputs, and edge instances. This volume of knowing The natural way qualified prospects to raised code framework and fewer bugs.
When debugging a concern, composing a failing test that reproduces the bug might be a powerful initial step. As soon as the check fails continually, you'll be able to deal with fixing the bug and look at your test pass when The problem is settled. This technique ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—encouraging you capture additional bugs, faster and even more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Option. But One of the more underrated debugging applications is simply stepping away. Taking breaks helps you reset your mind, decrease disappointment, and sometimes see the issue from a new perspective.
When you are far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this state, your brain becomes significantly less effective at issue-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for ten–15 minutes can refresh your focus. Lots of builders report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate within the background.
Breaks also assistance avert burnout, Specifically throughout longer debugging classes. Sitting before a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You may perhaps quickly discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you prior to.
For those who’re caught, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It may well come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it really brings about faster and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It gives your Mind space to breathe, improves your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you come upon is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can teach you some thing worthwhile when you make an effort to reflect and examine what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. After some time, you’ll begin to see designs—recurring problems or common mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a short generate-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your advancement journey. After all, several of the very best builders aren't those who write best code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer in your talent set. So up coming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, recall: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.